Using Loops
Loops are a fundamental concept in programming, enabling the execution of certain statements repeatedly as long as a given condition is met. They allow for efficient, compact code, handling repetitive tasks without manually duplicating instructions. Loops are particularly useful for:
- Searching through data structures,
- Repeatedly executing calculations,
- Implementing algorithms.
XenoGuard supports the most common types of loops:
- For Loop
- While
- Repeat Until
- For Each
These loops can be found in the Flow Control folder of the Programming group in XenoGuard.
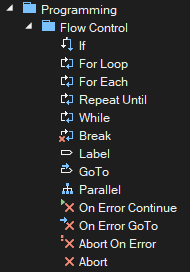
For Loop
The For loop in XenoGuard is a control structure enabling the repeated execution of a specific group of actions in a predefined sequence.

The For loop comprises five input parameters: an optional count variable (Identifier), start value (Start), comparison condition (Comparison), end value (End), and step size (Step). Actions within the loop are executed repeatedly as long as the comparison condition holds. After each iteration, typically the count variable is adjusted. The loop concludes when the condition is no longer met, continuing with subsequent actions.
While
A While loop in XenoGuard is a control structure that executes a sequence of actions repeatedly as long as a specified condition remains true. Its basic operation is as follows:
- Initial Condition Check: Before starting the loop, the specified condition is evaluated.
- Execution of Loop Content: If the condition evaluates to true, the set of actions within the loop is executed.
- Condition Re-evaluation: After executing the actions, the condition is checked again.
- Continuation or Termination: If the condition is still true, the loop repeats its actions. If not, the loop terminates, and the program resumes execution with the next statement after the loop.
It's crucial to modify the condition or related data within the loop to prevent infinite loops. This change should be designed such that the condition eventually becomes false, either after a certain number of iterations or due to specific circumstances.
Creating infinite loops is also possible, and it's important to use them cautiously. Here’s an illustrative example:
This structure ensures that the while loop in XenoGuard is utilized effectively, avoiding unintended infinite looping while allowing repetitive task execution based on dynamic conditions.
Repeat Until
The Repeat Until loop is a control structure that repeatedly executes a set of actions until a specified condition becomes true. Unlike other loop forms such as the While loop, the Repeat Until loop executes the actions first before checking the condition. This guarantees that the actions within the loop are executed at least once, irrespective of the initial truth value of the condition.
Here's how it works:
- Execute Actions: The loop first executes the actions within its branch.
- Condition Check: After the actions are executed, the condition is evaluated.
- Loop Continuation or Termination: If the condition evaluates to false, the actions are executed again. This process repeats until the condition becomes true. Once true, the loop terminates, and the program proceeds with the actions that follow the loop.
This structure ensures that the Repeat Until loop in XenoGuard provides a reliable way to perform actions at least once and then continue based on a specific condition.
The Repeat Until ActionStep in XenoGuard includes three additional parameters that modify its behavior:
-
Loop Delay: This parameter allows you to set a waiting period, in milliseconds, between iterations of the loop. This feature enables the programming of deliberate delays within the loop.
-
Max Repetitions: This parameter specifies the maximum number of times the loop will execute before it terminates, regardless of the termination condition. It's useful for ensuring that the loop doesn't run indefinitely.
-
Condition Stability Time: Expressed in milliseconds, this parameter determines how long the termination condition's value must remain constant before the loop exits. If the termination condition changes during this time, the loop continues until the condition stabilizes again for the entire specified duration. Mathematically, this can be expressed as follows:
Given:
- C(t): The value of the termination condition at time t
- T: Condition Stability Time in milliseconds
The loop stops when, for all t1 and t2 within an interval of T milliseconds, C(t1) = C(t2) holds true.
The figure shown above illustrates an example of using the Condition Stability Time parameter in the Repeat Until action. In this scenario, the action monitors the File Count result parameter from the Directory Get Files. The loop terminates if no new files are found in the C:\Temp\ folder over a period of T = 5000 ms, provided that at least one file is present. This setup is especially beneficial when another program or user is modifying the contents of the folder in the background, allowing the script to wait before further processing the files.
For Each
The For Each action in XenoGuard iterates through result lists. Details follow in the next section.
Exiting Loops
The Break action in XenoGuard allows for early termination of loops. If executed within a loop (For or While), it immediately ends the loop, proceeding with the script's next statement. This is effective when further iteration is unnecessary or a specific condition has been met.
In summary, XenoGuard's loop structures provide versatile tools for efficiently managing repetitive actions and workflows.